We can find five basic different types of events:
1-List Events
2-List Item Events
3-List Email Events
4-Web Events
5-List Workflow Events
Because we are going to reproduce some kind of event in one of the lists we will need to choose "List Item Events". The event source will be Announcements, and the handle event will be called (...).
In order to do this we will have to override ItemAdded by calling this method:
public override void ItemAdded(SPItemEventProperties properties)
It is important to remember to use using(...) to avoid memory leakings, in this way, Sharepoint will be able to dispose the objects properly. As you probably don't know Sharepoint doesn't dispose some of the objects properly (ie: SPWeb).
Let's go to start building a new project. Go to Visual Studio 2010, clik on File->New Project->Sharepoint->2010->Event Receiver.
Name the project with AddingDateTimeToAnnoucement an follow the steps in the scheenshots.
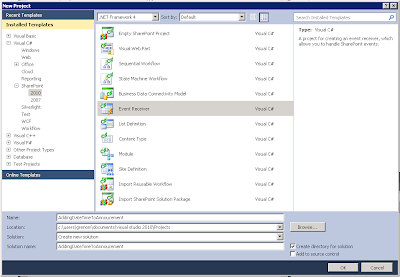
Select the solution as Sand box solution and pointed to the right URL
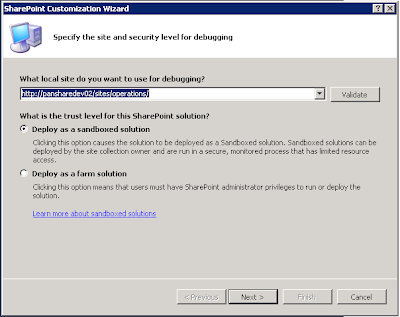
Click on Next and choose "List Item Events" and in event source "Announcements" and in Handle the following events tick "An item was added".
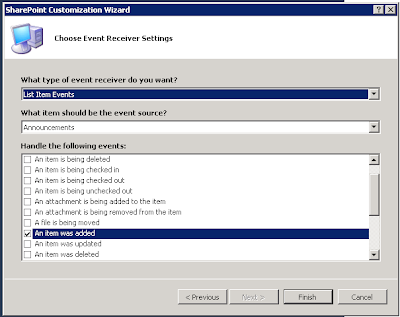
Rename the .cs class to AnnoucementEventReceiver.cs, go inside and rename the class as well with the same name. On features rename the feature1 to AnnoucementEventReceiverFeature. Go to "Elements.xml" and be sure that the xml code looks like this:
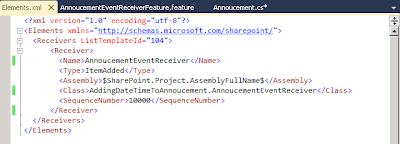
Be sure than in Features you choose Site on the "Scope:" so it should look something like this: (There is a mistake in the image "Annoucements.cs" should be "AnnoucementEventReceiver.cs")
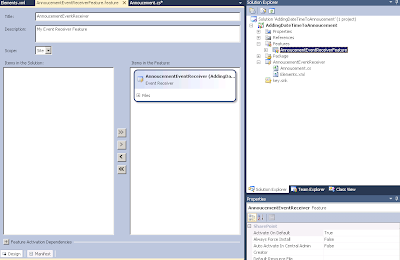
Copy and paste the following code into your class:
using System;
using System.Security.Permissions;
using Microsoft.SharePoint;
using Microsoft.SharePoint.Security;
using Microsoft.SharePoint.Utilities;
using Microsoft.SharePoint.Workflow;
namespace AddingDateTimeToAnnoucement
{
///
/// List Item Events
///
public class AnnoucementEventReceiver : SPItemEventReceiver
{
///
/// An item was added.
///
public override void ItemAdded(SPItemEventProperties properties)
{
using (SPSite _spsCurrentSite = new SPSite(properties.SiteId))
{
using (SPWeb _spwCurrentWeb = _spsCurrentSite.RootWeb )
{
SPListCollection _splListCollection = _spwCurrentWeb.Lists;
foreach (SPList item in _splListCollection)
{
if (item.Title == "Announcements")
{
SPListItemCollection _splListOfAnnouncements = item.Items;
foreach (SPListItem _splItem in _splListOfAnnouncements)
{
if (_splItem["Title"].ToString() == properties.ListItem["Title"].ToString())
{
_splItem["Title"] = properties.ListItem["Title"].ToString() + DateTime.Now.ToString();
_splItem.Update();
}
}
}
}
}
}
base.ItemAdded(properties);
}
}
}
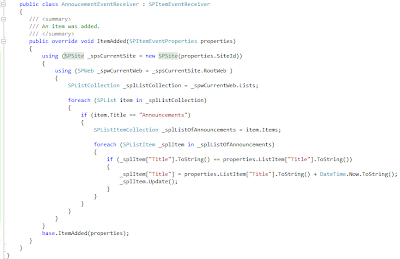
You should be able to press F5 now. Go to "Lists" click on "Annoucements" create a new one and you will see how the title change with the title + the current date.
Enjoy
No comments:
Post a Comment